Butter Knife
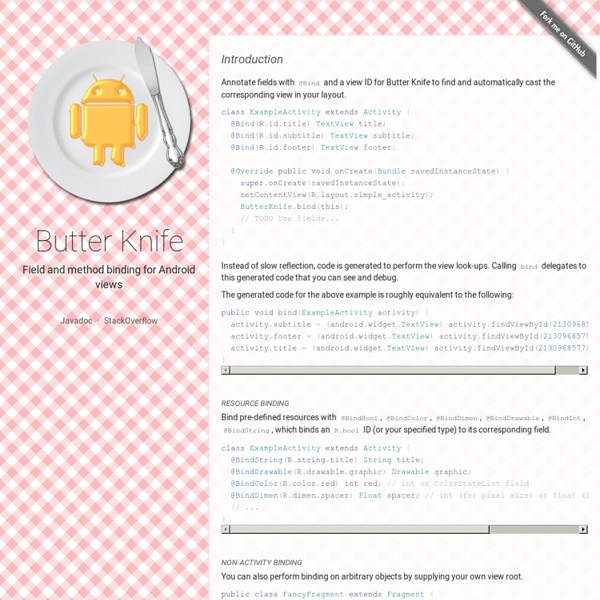
Introduction Annotate fields with @Bind and a view ID for Butter Knife to find and automatically cast the corresponding view in your layout. class ExampleActivity extends Activity { @Bind(R.id.title) TextView title; @Bind(R.id.subtitle) TextView subtitle; @Bind(R.id.footer) TextView footer; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.simple_activity); ButterKnife.bind(this); // TODO Use fields... }} Instead of slow reflection, code is generated to perform the view look-ups. The generated code for the above example is roughly equivalent to the following: public void bind(ExampleActivity activity) { activity.subtitle = (android.widget.TextView) activity.findViewById(2130968578); activity.footer = (android.widget.TextView) activity.findViewById(2130968579); activity.title = (android.widget.TextView) activity.findViewById(2130968577);} Resource Binding Non-Activity Binding Other provided binding APIs: View Lists Bonus Maven
Robolectric
OkHttp
Overview HTTP is the way modern applications network. It’s how we exchange data & media. Doing HTTP efficiently makes your stuff load faster and saves bandwidth. OkHttp is an HTTP client that’s efficient by default: HTTP/2 support allows all requests to the same host to share a socket. OkHttp perseveres when the network is troublesome: it will silently recover from common connection problems. Using OkHttp is easy. OkHttp supports Android 2.3 and above. Examples Get a URL This program downloads a URL and print its contents as a string. OkHttpClient client = new OkHttpClient(); String run(String url) throws IOException { Request request = new Request.Builder() .url(url) .build(); Response response = client.newCall(request).execute(); return response.body().string();} Post to a Server This program posts data to a service. Download ↓ v3.4.1 JAR You'll also need Okio, which OkHttp uses for fast I/O and resizable buffers. Maven Gradle compile 'com.squareup.okhttp3:okhttp:3.4.1' Contributing License
Retrofit
Introduction Retrofit turns your REST API into a Java interface. public interface GitHubService { @GET("/users/{user}/repos") List<Repo> listRepos(@Path("user") String user);} The RestAdapter class generates an implementation of the GitHubService interface. RestAdapter restAdapter = new RestAdapter.Builder() .setEndpoint(" .build(); GitHubService service = restAdapter.create(GitHubService.class); Each call on the generated GitHubService makes an HTTP request to the remote webserver. List<Repo> repos = service.listRepos("octocat"); Use annotations to describe the HTTP request: URL parameter replacement and query parameter support Object conversion to request body (e.g., JSON, protocol buffers) Multipart request body and file upload API Declaration Annotations on the interface methods and its parameters indicate how a request will be handled. Request Method Every method must have an HTTP annotation that provides the request method and relative URL. @GET("/users/list") Download
Picasso
Introduction Images add much-needed context and visual flair to Android applications. Picasso allows for hassle-free image loading in your application—often in one line of code! Picasso.with(context).load(" Many common pitfalls of image loading on Android are handled automatically by Picasso: Handling ImageView recycling and download cancelation in an adapter. Features Adapter Downloads Adapter re-use is automatically detected and the previous download canceled. @Override public void getView(int position, View convertView, ViewGroup parent) { SquaredImageView view = (SquaredImageView) convertView; if (view == null) { view = new SquaredImageView(context); } String url = getItem(position); Picasso.with(context).load(url).into(view);} Image Transformations Transform images to better fit into layouts and to reduce memory size. Picasso.with(context) .load(url) .resize(50, 50) .centerCrop() .into(imageView) Place Holders Resource Loading Debug Indicators
Gradle, please
Related:
Related: