Understanding Ember.Object
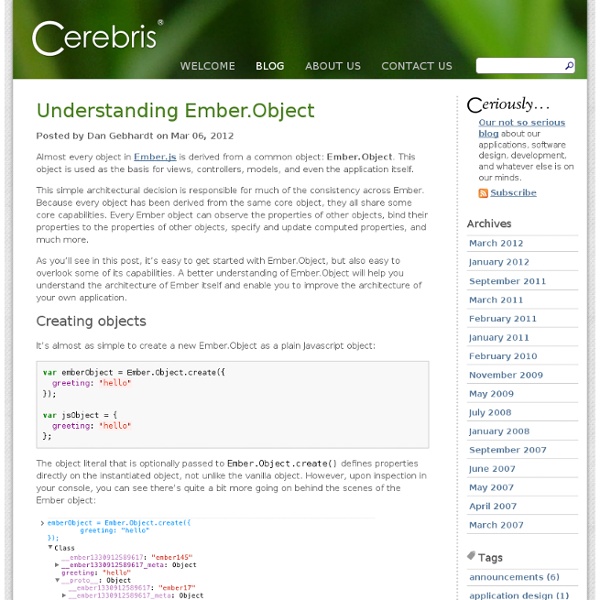
Almost every object in Ember.js is derived from a common object: Ember.Object. This object is used as the basis for views, controllers, models, and even the application itself. This simple architectural decision is responsible for much of the consistency across Ember. Because every object has been derived from the same core object, they all share some core capabilities. Every Ember object can observe the properties of other objects, bind their properties to the properties of other objects, specify and update computed properties, and much more. As you'll see in this post, it's easy to get started with Ember.Object, but also easy to overlook some of its capabilities. Creating objects It's almost as simple to create a new Ember.Object as a plain Javascript object: The object literal that is optionally passed to Ember.Object.create() defines properties directly on the instantiated object, not unlike the vanilla object. Extending classes Now, let's instantiate a person with create():
Tutorials
Web App Code Lab
Attention! Be sure to check the errata documention for important updates and a list of currently broken features. Introduction This codelab covers the techniques and design fundamentals required to create modern, 'lick-able' web applications. Using an MVC frameworkMaking cross-domain requests and handling JSON dataCreating user interfaces & experiences that are action oriented and app-likeEnabling offline experiences Prerequisites Before you begin, make sure you've downloaded the required codelab files from that you have a working development environment, and a working web server. How To Proceed Each exercise has it's own folder (for example /exercise1/). For each exercise, it's recommended that you start with the working version provided in the exercise folder to ensure that you're always starting from a known position, and that a mistake in a previous exercise doesn't pop up in a later exercise. Exercise 1 - Boiler plate Exercise 2.1 (js/app.js)
Ember.js tutorial for beginners « ephptutorial
There are some tasks that are common to every web application. For example, taking data from the server, rendering it to the screen, then updating that information when it changes. Since the tools provided to do this by the browser are quite primitive, you end up writing the same code over and over. Because we’ve built dozens of applications ourselves, we’ve gone beyond the obvious low-level event-driven abstractions, eliminating much of the boilerplate associated with propagating changes throughout your application, and especially into the DOM itself. To help manage changes in the view, Ember.js comes with a templating engine that will automatically update the DOM when the underlying objects change. As with any templating system, when the template is initially rendered, it will reflect the current state of the person. Since web applications evolved from web pages, which were nothing more than static documents, browsers give you just enough rope to hang yourself with. Child Views delegated
Writing an Ember.js App From Scratch (Part 2) | Drew Schrauf
In part 1 of this tutorial we began to make a very simple todo app which used Ember to handle all of the logic. If you haven't read it yet, check it out! Our little todo app is now displaying a couple of prepopulated Todo items with a little counter at the bottom that shows the number of incomplete items. Obviously, a todo app isn't very useful if you can't add your own items to it so that's what we'll dive into next. As before, our controller is where all of our business logic should be sitting so we'll start by adding a method to our controller which will add a new item to our list. The function createTodo that we've defined here doesn't really do anything special. If you remember the Ember.Checkbox we used in part 1 to bind a Todo's properties to a view, you can probably guess at where we're going next. All we've done here is override the insertNewline method of Ember's TextField to make it call the createTodo method we defined earlier if the user has entered a value. …into this:
Flame on! A beginner's guide to Ember.js
Sophisticated JavaScript applications can be found all over the place these days. As these applications become more and more complex, it's no longer acceptable to have a long chain of jQuery callback statements, or even distinct functions called at various points through your application. This has led to JavaScript developers learning what traditional software programmers have known for decades: organization and efficiency are important and can make the difference between an application that performs great and one that doesn't. One of the most commonly used architecture patterns to achieve this organization and efficiency is known as Model View Controller (or MVC). This pattern encourages developers to separate distinct parts of their application into pieces that are more manageable. In this tutorial you'll become more familiar with the basics of Ember.js as you build a working Twitter timeline viewer. Ember has only one dependency—jQuery. Application Models Views Handlebars Controllers <!
Related:
Related: