Processing.js
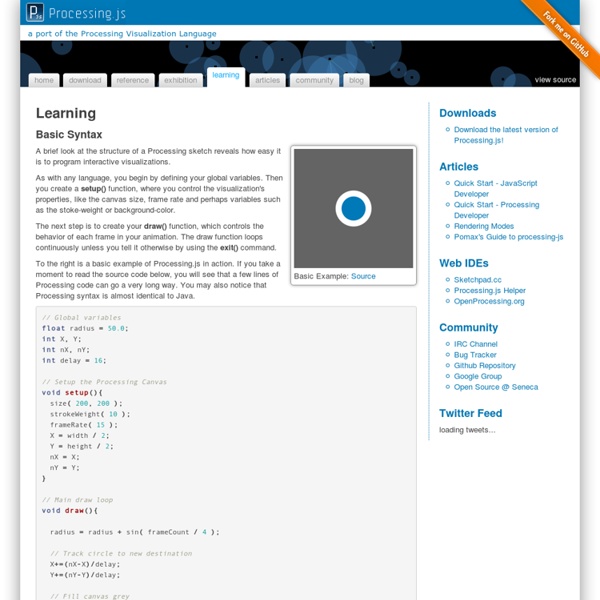
Basic Syntax A brief look at the structure of a Processing sketch reveals how easy it is to program interactive visualizations. As with any language, you begin by defining your global variables. The next step is to create your draw() function, which controls the behavior of each frame in your animation. To the right is a basic example of Processing.js in action. // Global variablesfloat radius = 50.0;int X, Y;int nX, nY;int delay = 16; // Setup the Processing Canvasvoid setup(){ size( 200, 200 ); strokeWeight( 10 ); frameRate( 15 ); X = width / 2; Y = height / 2; nX = X; nY = Y; } // Main draw loopvoid draw(){ radius = radius + sin( frameCount / 4 ); // Track circle to new destination X+=(nX-X)/delay; Y+=(nY-Y)/delay; // Fill canvas grey background( 100 ); // Set fill-color to blue fill( 0, 121, 184 ); // Set stroke-color white stroke(255); // Draw circle ellipse( X, Y, radius, radius ); } // Set circle's next destinationvoid mouseMoved(){ nX = mouseX; nY = mouseY; } Using Processing
Workshop / Chrome Experiments
Unfortunately, either your web browser or your graphics card doesn't support WebGL. We recommend you try it again with Google Chrome.
Mercurial SCM
skilldrick.co.uk » A brief introduction to closures
In this post, I’m going to attempt to explain what closures are and how to use them. Many modern (and some not-so-modern) programming languages contain support for closures, but for the purposes of this article I’m going to be using JavaScript. I’ve chosen JavaScript for a few reasons: Ubiquity: If you have a web browser then you have a JavaScript interpreterSimplicity: JavaScript is conceptually a fairly simple language (especially if you limit yourself to its Good Parts), compared to other dynamic scripting languages such as Python and RubyFamiliarity: If you’ve used any of the C family of languages (e.g. C++, Java or C#) then JavaScript will look fairly familiar. There may be some differences between languages with the mechanics, but deep down, closures are the same across all languages which allow them, so if you can understand the concept in JavaScript, you’ll understand it in any capable language. function f() { alert('f called!') function () { alert('I have no name'); }
Introduction to Circos, Features and Uses // CIRCOS Circular Genome Data Visualization
Apache CXF -- Index
Understanding JavaScript Closures | JavaScript, JavaScript
In JavaScript, a closure is a function to which the variables of the surrounding context are bound by reference. Every JavaScript function forms a closure on creation. In a moment I’ll explain why and walk through the process by which closures are created. Then I’ll address some common misconceptions and finish with some practical applications. Lexical Scope The word lexical pertains to words or language. Consider the following example: Function inner is physically surrounded by function outer which in turn is wrapped by the global context. global outer inner The outer lexical scope of any given function is defined by its ancestors in the lexical hierarchy. VariableEnvironment The global object has an associated execution context. [Note in EcmaScript 3, the VariableEnvironment of a function was known as the ActivationObject - which is also the term I used in some older articles] We could represent the VariableEnvironment with pseudo-code… The [[scope]] property Dispelling the Myths Myth 1.
Java Business Integration
Java Business Integration (JBI) is a specification developed under the Java Community Process (JCP) for an approach to implementing a service-oriented architecture (SOA). The JCP reference is JSR 208 for JBI 1.0 and JSR 312 for JBI 2.0. JBI is built on a Web Services model and provides a pluggable architecture for a container that hosts service producer and consumer components. Services connect to the container via binding components (BC) or can be hosted inside the container as part of a service engine (SE). The services model used is Web Services Description Language 2.0. The central message delivery mechanism, the normalized message router (NMR), delivers normalized messages via one of four Message Exchange Patterns (MEPs), taken from WSDL 2.0: In-Only: A standard one-way messaging exchange where the consumer sends a message to the provider that provides only a status response.Robust In-Only: This pattern is for reliable one-way message exchanges. JBI implementations[edit] Books[edit]
Head JS :: The only script in your HEAD
Related:
Related: