74 Quality Ruby on Rails Resources and Tutorials
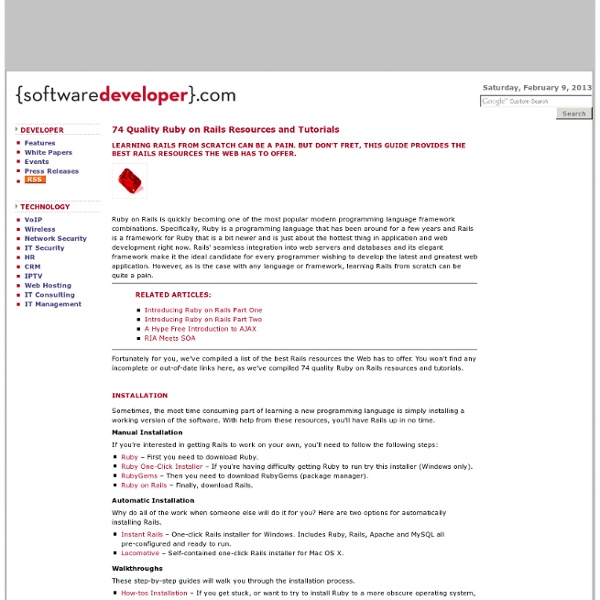
Learning Rails from scratch can be a pain. But don't fret, this guide provides the best Rails resources the Web has to offer. Ruby on Rails is quickly becoming one of the most popular modern programming language framework combinations. Related Articles: Fortunately for you, we've compiled a list of the best Rails resources the Web has to offer. Installation Sometimes, the most time consuming part of learning a new programming language is simply installing a working version of the software. Manual Installation If you're interested in getting Rails to work on your own, you'll need to follow the following steps: Ruby – First you need to download Ruby. Why do all of the work when someone else will do it for you? Instant Rails – One-click Rails installer for Windows. These step-by-step guides will walk you through the installation process. How-tos Installation – If you get stuck, or want to try to install Ruby to a more obscure operating system, look here. Tutorials Beginner Tutorials Try Ruby!
Related: